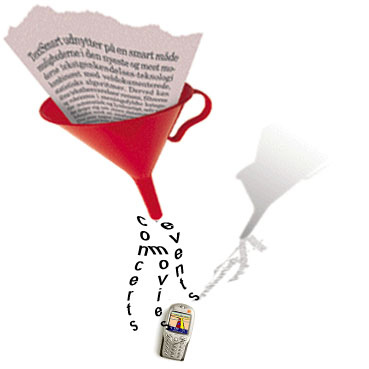
JavaScript&JQuery-網站互動設計程式進化之道-Filtering, Searching, & Sorting
學習筆記(11) - Filtering, Searching, & Sorting
一名最好的醫生,就在於能最大限度地克制自己的感情,不讓對某些不可挽回的事情的悔恨,干擾完成未來任務的決心 - 保羅·海澤
引言
原文書名:JavaScript & JQuery: Interactive Front-End Web Development
作者:Jon Duckett
譯者:謝銘倫
出版社:碁峰
出版日期:2017/02/24
十二、Filtering, Searching, & Sorting
Filtering
1 | // .forEach() .push() |
動態資料過濾
1 | // Dynamically Filtering an Array |
影像過濾(data-tags)
1 | // 處理標記data-tags |
Searching
1 | // Searching cache = []; |
Sorting
1 | //Sorting data-sort |