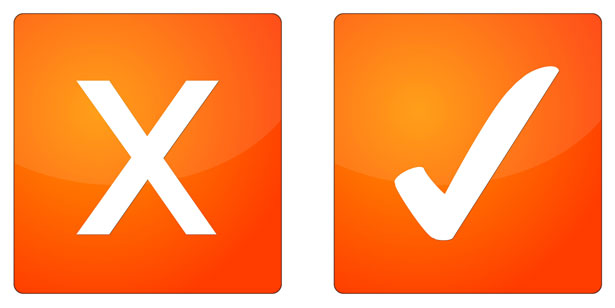
JavaScript&JQuery-網站互動設計程式進化之道-Form Enhancement & Validation
學習筆記(12) - Form Enhancement & Validation
少年人須有老成之識見,老成人須有少年之襟懷 - 張潮《幽夢影》
引言
原文書名:JavaScript & JQuery: Interactive Front-End Web Development
作者:Jon Duckett
譯者:謝銘倫
出版社:碁峰
出版日期:2017/02/24
十三、Form Enhancement & Validation
表單驗證
1 | // JavaScript validation of subscription form. |
1 | // ------------------------------------------------------------------------- |
1 | // ------------------------------------------------------------------------- |
1 | // ------------------------------------------------------------------------- |
1 | // ------------------------------------------------------------------------- |