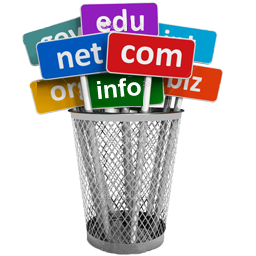
JavaScript&JQuery-網站互動設計程式進化之道-Content Panels
學習筆記(10) - Content Panels
堅韌是成功的一大要素,只要在門上敲得夠久、夠大聲,終會把人喚醒的
引言
原文書名:JavaScript & JQuery: Interactive Front-End Web Development
作者:Jon Duckett
譯者:謝銘倫
出版社:碁峰
出版日期:2017/02/24
十一、Content Panels
摺疊收合:bootstrap-Collapse
1 | //jQuery accordion |
頁籤面板:bootstrap-Navs
1 | //jQuery tab-list |
懸浮視窗:bootstrap-Modal
1 | //jQuery modal |
滑動面板-bootstrapcarousel
1 | //jQuery slider |
相片檢視
1 | //jQuery thumbnail |