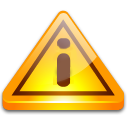
JavaScript&JQuery-網站互動設計程式進化之道-Error Handling & Debugging
學習筆記(9) - Error Handling & Debugging
堅韌是成功的一大要素,只要在門上敲得夠久、夠大聲,終會把人喚醒的
引言
原文書名:JavaScript & JQuery: Interactive Front-End Web Development
作者:Jon Duckett
譯者:謝銘倫
出版社:碁峰
出版日期:2017/02/24
十、Error Handling & Debugging
ERROR物件
- Error:所有Error物件的樣本
- SyntaxError:語法不正確
- ReferenceError:變數不存在
- URIError:未正確使用URI函式(字元未跳脫 / ? & # : ; )
- TypeError:資料為非預期的型別(不存在的物件或方法)
- RangeError:數值超出定義範圍
- EvalError:未正確使用eval()函式
- NaN:運算使用了非數值
Console
1 | //console.log() |
Add Conditional Breakpoint
1 | if (area < 100) { |
例外處理
1 | if (response) { |